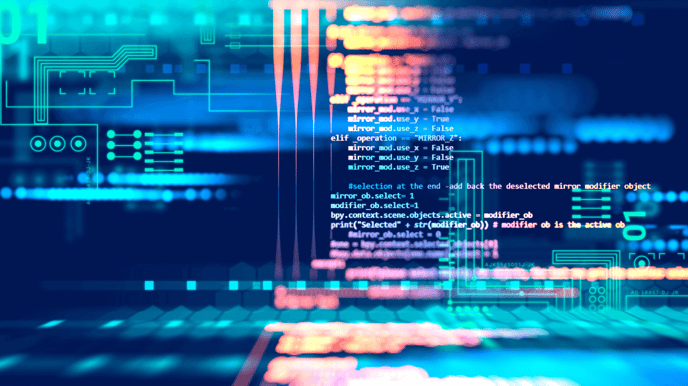
Roblox Lua Scripting: Getting Started with Coding
Posted by Steve Davies October 11th, 2023
Roblox is a unique platform in the wide world of game development that encourages gamers to develop their own games rather than merely playing them. All this creativity is powered by Lua scripting which is quite powerful and flexible and enables the Roblox developers to translate their thoughts into reality. If you are looking to enter the world of gaming development or it is simply your curiosity directed towards the programming world in Roblox then, this guide shall take you by hand in introducing yourself to the fundamentals of Lua scripting for Roblox.
UNDERSTANDING ROBLOX LUA SCRIPTING
What is Lua?
Roblox’s core programming language – Lua – is well-known as easy and flexible. It is lightweight and simple to learn which makes it best for novice users. For instance, it is used by the developers of Roblox for creating interactive game elements, controlling the behavior of in-game objects, and defining game mechanics.
The Roblox Studio
First, let us take a look at Roblox Studio – an official development environment that can be used in order to create various scripts using Lua. The place here you will create your own game, including tools for Lua scripting. This studio has an easy-to-use and visible to users for new and professional programmers.
SETTING UP YOUR WORKSPACE
Installing Roblox Studio
You would have to install Roblox Studio beforehand if you will work with it. To install studio visit the Roblox official Website and Download Studio, and follow its installation procedure.
Creating a Roblox Account
You will require a Roblox account to use the Studio. Create an account on the Roblox website if you don’t have one. This is absolutely free, and it leads into a thriving network of programmers and game lovers.
Starting a New Project
Once you're logged in, open Roblox Studio and start a new project. Choose a template or begin from scratch—this is where your creative journey begins.
THE BASICS OF ROBLOX LUA SCRIPTING
Understanding Objects and Instances
In Roblox, everything is an object, and objects are instances of classes. For example, a part of the game environment, like a brick, is an object, and each brick you place is an instance of that object. Lua scripting allows you to control the properties and behaviors of these objects.
Hello World in Lua
The traditional starting point for any programming language is the "Hello World" program. In Lua, it's as simple as:
print("Hello, World!")
In Roblox Studio, you can run this script to see the output in the output window. This simple example illustrates the basic syntax of Lua scripting in Roblox.
Variables and Data Types
Like any programming language, Lua uses variables to store and manipulate data. Understanding data types is crucial. In Lua, common data types include strings, numbers, and booleans. Here's a quick example:
local playerName = "John"
local playerScore = 100
local isGameOver = false
In this example, ‘playerName’ is a string, ‘playerScore’ is a number, and ‘isGameOver’ is a boolean.
CONTROLLING FLOW WITH CONTROL STRUCTURES
Conditional Statements
Conditional statements allow your script to make decisions based on certain conditions. The ‘if’, ‘else’, and ‘elseif' statements are fundamental to controlling the flow of your code.
local playerHealth = 75
if playerHealth > 50 then
print("Player is in good health.")
else
print("Player needs healing.")
end
This script checks the player's health and prints a message based on the condition.
Loops
Loops are essential for executing a block of code repeatedly. The for and while loops are commonly used in Lua scripting.
for i = 1, 5 do
print("Count: " .. i)
end
This script uses a for loop to print the numbers 1 through 5.
FUNCTIONS AND MODULARITY
Defining Functions
Functions are blocks of reusable code that perform a specific task. They enhance code readability and maintainability.
function greetPlayer(playerName)
print("Hello, " .. playerName .. "!")
end
greetPlayer("Alex")
In this example, the ‘greetPlayer’ function takes a ‘playerName’ parameter and prints a greeting.
Libraries and Modules
Lua supports the creation of libraries and modules to organize and reuse code across different parts of your game. This promotes modularity and efficient code management.
local myLibrary = {}
function myLibrary.doubleNumber(num)
return num * 2
end
return myLibrary
This simple module doubles a given number and can be reused in different scripts.
EVENT HANDLING AND INTERACTIVITY
Understanding Events
Events are crucial in game development as they allow your script to respond to actions or changes in the game environment. Common events include player input, collisions, and time-based events.
local button = script.Parent
button.MouseButton1Click:Connect(function()
print("Button clicked!")
end)
This script detects when a button is clicked and prints a message.
User Interface (UI) Elements
Lua scripting in Roblox extends beyond game mechanics to include the creation of user interfaces. You can use scripts to control the behavior of buttons, text boxes, and other UI elements.
local player = game.Players.LocalPlayer
local playerGui = player.PlayerGui
local frame = Instance.new("Frame")
frame.Size = UDim2.new(0, 200, 0, 100)
frame.Position = UDim2.new(0.5, -100, 0.5, -50)
frame.BackgroundColor3 = Color3.new(1, 1, 1)
frame.Parent = playerGui
This script creates a simple frame in the player's GUI.
DEBUGGING AND TROUBLESHOOTING
Using the Output Window
The Output window in Roblox Studio is your best friend when it comes to debugging. If your script isn't working as expected, use print statements to output information and identify potential issues.
Reading Error Messages
Don't be afraid of error messages—they are your guides to identifying and fixing problems in your code. Take the time to read and understand what the error is telling you.
ADVANCING YOUR SKILLS
Roblox Developer Hub
The Roblox Developer Hub is an invaluable resource for developers of all skill levels. It offers documentation, tutorials, and a community forum where you can seek help and share your experiences.
Online Communities
Joining online communities, such as the Roblox Developer Forum, allows you to connect with other developers, share your work, and learn from experienced members of the Roblox community.
Experiment and Iterate
The best way to learn is by doing. Experiment with different scripts, modify existing ones, and see how changes impact your game. Don't be afraid to make mistakes—they're part of the learning process.
Conclusion
Embarking on your Roblox Lua scripting journey opens a world of possibilities for creativity and innovation. Whether you're crafting immersive games, interactive experiences, or dynamic user interfaces, Lua scripting empowers you to bring your ideas to life within the Roblox universe. Remember, the key to mastering Lua is practice and a willingness to explore. So, dive into Roblox Studio, experiment with scripts, and let your creativity flourish in the dynamic world of game development.